MinGW 2Dグラフィックス
2Dグラフィックス
MinGW で2次元グラフィックスを出力する方法は、いろいろありますが、ここでは、WinBGIm (Borland BGI Graphics emulation) をソースからコンパイルして、導入します。そして、ライフゲームの結果をグラフィックスで表示します。
WinBGIm のインストール
WinBGIm のソースプログラムは、インターネット上の、http://www.codecutter.net/tools/winbgim/ から、Download The WinBGIm 6.0 (Nov 2005) source code にある、”here” をクリックして、WinBGIm6_0_Nov2005_src.zip をダウンロードします。
ZIP ファイルを解凍し、winbgim.h, graphics.h の302行目にある、int right=0, int right=INT_MAX を int top=0, int right=INT_MAX に変更します。そして、make コマンドによりコンパイルを実行します。
以下のファイルを MinGW のインストールディレクトリにコピーします。
- winbgim.h -> MinGW/include
- graphics.h -> MinGW/include
- libbgi.a -> MinGW/lib
ライフゲームプログラム
ライフゲームの結果をグラフィック出力するソースプログラムを以下に示します。
#include <stdio.h>
#include <windows.h>
#include <graphics.h> // g++ life2g.c -lbgi -lgdi32 -lcomdlg32 -luuid -loleaut32 -lole32
#define MAXSTEP 600 // Step
#define BMX 79 // Max x size of board
#define BMY 48 // Max y size of board
int b[BMX+2][BMY+2]; // Board Empty:0 Marked:3
void inputboard() {
int x;
int y;
for(y = 0; y < BMY+2; y++) {
for(x = 0; x < BMX+2; x++) {
b[x][y] = 0;
}
}
b[40][14] = 3;
b[40][15] = 3;
b[40][16] = 3;
b[41][14] = 3;
b[39][15] = 3;
}
void outputboard(int i) {
int x;
int y;
char buf[128];
sprintf(buf, "Step : %5d", i);
setcolor(15);
outtextxy(7, BMY * 7 + 10, buf);
}
void initscreen() {
initwindow(600, 400, "Life game");
}
int countalive(int x, int y) {
int cal = 0;
if(b[x-1][y-1] > 1) cal ++;
if(b[x-1][y] > 1) cal ++;
if(b[x-1][y+1] > 1) cal ++;
if(b[x][y-1] > 1) cal ++;
if(b[x][y+1] > 1) cal ++;
if(b[x+1][y-1] > 1) cal ++;
if(b[x+1][y] > 1) cal ++;
if(b[x+1][y+1] > 1) cal ++;
return cal;
}
void nextstep() {
int x;
int y;
int cal;
for(y = 1; y <= BMY; y++) {
for(x = 1; x <= BMX; x++) {
cal = countalive(x, y);
if(b[x][y] == 0) {
if(cal == 3) b[x][y] = 1;
} else if(b[x][y] == 3) {
if(cal < 2) b[x][y] = 2;
if(cal > 3) b[x][y] = 2;
} else {
printf("Unknown code of board[%d][%d]\n", x, y);
}
}
}
for(y = 1; y <= BMY; y++) {
for(x = 1; x <= BMX; x++) {
if(b[x][y] == 1) {
b[x][y] = 3;
setfillstyle(SOLID_FILL, 14);
setcolor(14);
fillellipse(x*7, y*7, 3, 3);
}
if(b[x][y] == 2) {
b[x][y] = 0;
setfillstyle(SOLID_FILL, 0);
setcolor(0);
fillellipse(x*7, y*7, 3, 3);
}
}
}
}
int main() {
int i = 0;
inputboard();
initscreen();
for(i = 1; i <= MAXSTEP; i++) {
outputboard(i);
nextstep();
}
return 0;
}
ソースプログラムを life2g.c とした場合、コンパイルは以下のように実行してください。
> g++ life2g.c -lbgi -lgdi32 -lcomdlg32 -luuid -loleaut32 -lole32
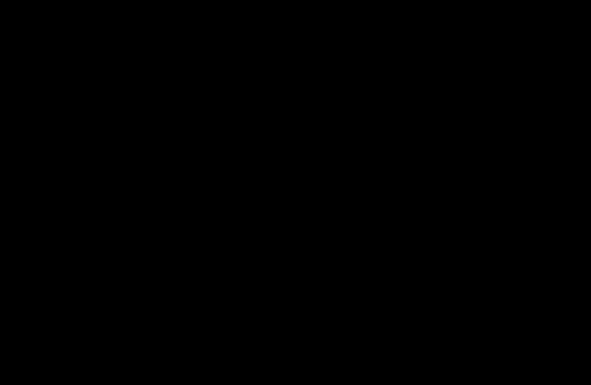